Definition
Streamlize objects by providing an interface to encapsulate a request and make the interface implemented by subclasses in order to parameterize the clients.Where to use & benefits
- One action can be represented in many ways, like drop-down menu, buttons and popup menu.
- Need a callback function, i.e., register it somewhere to be called later.
- Specify and execute the request at different time
- Need to undo an action by storing its states for later retrieving.
- Decouple the object with its trigger
- Easily to be extensible by not touching the old structure.
- Related patterns include
Intent
01- Encapsulate a request as an object, thereby letting you parameterize clients with different requests, queue or log requests, and support undoable operations.
- Promote “invocation of a method on an object” to full object status
- An object-oriented callback
Problem
02Need to issue requests to objects without knowing anything about the operation being requested or the receiver of the request.
Intent
- encapsulate a request in an object- allows the parameterization of clients with different requests
- allows saving the requests in a queue
Implementation
The idea and implementation of the Command design pattern is quite simple, as we will see in the diagram below, needing only few extra classes implemented.
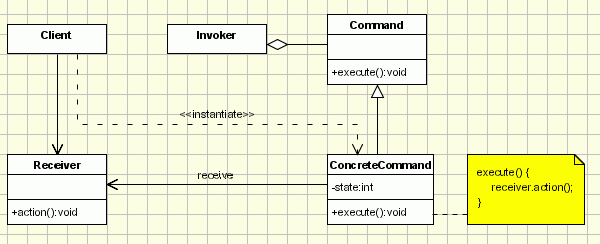
The classes participating in the pattern are:
- Command - declares an interface for executing an operation;
- ConcreteCommand - extends the Command interface, implementing the Execute method by invoking the corresponding operations on Receiver. It defines a link between the Receiver and the action.
- Client - creates a ConcreteCommand object and sets its receiver;
- Invoker - asks the command to carry out the request;
- Receiver - knows how to perform the operations;
The Client asks for a command to be executed. The Invoker takes the command, encapsulates it and places it in a queue, in case there is something else to do first, and the ConcreteCommand that is in charge of the requested command, sending its result to the Receiver.
Here is a sample code of a classic implementation of this pattern for placing orders for buying and selling stocks:
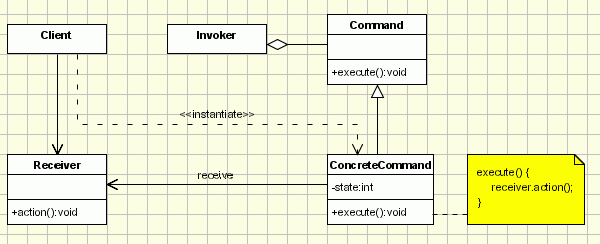
The classes participating in the pattern are:
- Command - declares an interface for executing an operation;
- ConcreteCommand - extends the Command interface, implementing the Execute method by invoking the corresponding operations on Receiver. It defines a link between the Receiver and the action.
- Client - creates a ConcreteCommand object and sets its receiver;
- Invoker - asks the command to carry out the request;
- Receiver - knows how to perform the operations;
The Client asks for a command to be executed. The Invoker takes the command, encapsulates it and places it in a queue, in case there is something else to do first, and the ConcreteCommand that is in charge of the requested command, sending its result to the Receiver.
Here is a sample code of a classic implementation of this pattern for placing orders for buying and selling stocks:
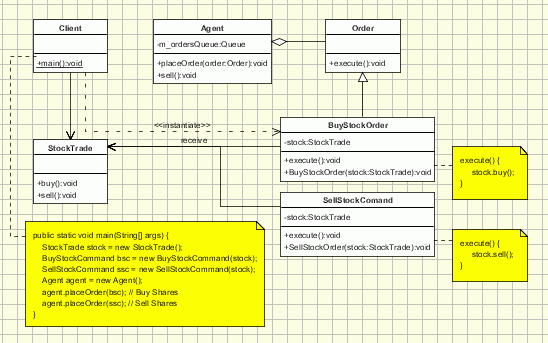
The client creates some orders for buying and selling stocks (ConcreteCommands). Then the orders are sent to the agent (Invoker).The agent takes the orders and place them to the StockTrade system (Receiver). The agent keeps an internal queue with the order to be placed. Let's assume that the StockTrade system is closed each Monday, but the agent accepts orders, and queue them to be processed later on.
public interface Order { public abstract void execute ( ); } // Receiver class. class StockTrade { public void buy() { System.out.println("You want to buy stocks"); } public void sell() { System.out.println("You want to sell stocks "); } } // Invoker. class Agent { private m_ordersQueue = new ArrayList(); public Agent() { } void placeOrder(Order order) { ordersQueue.addLast(order); order.execute(ordersQueue.getFirstAndRemove()); } } //ConcreteCommand Class. class BuyStockOrder implements Order { private StockTrade stock; public BuyStockOrder ( StockTrade st) { stock = st; } public void execute( ) { stock . buy( ); } } //ConcreteCommand Class. class SellStockOrder implements Order { private StockTrade stock; public SellStockOrder ( StockTrade st) { stock = st; } public void execute( ) { stock . sell( ); } } // Client public class Client { public static void main(String[] args) { StockTrade stock = new StockTrade(); BuyStockOrder bsc = new BuyStockOrder (stock); SellStockOrder ssc = new SellStockOrder (stock); Agent agent = new Agent(); agent.placeOrder(bsc); // Buy Shares agent.placeOrder(ssc); // Sell Shares } } |
Applicability & Examples
This is another of the data-driven pattern. The client invokes a particular module using a command. The client passes a request, this request gets propagated as a command. The command request maps to particular modules. According to the command, a module is invoked.
This pattern is different from the Chain of Responsibility in a way that, in the earlier one, the request passes through each of the classes before finding an object that can take the responsibility. The command pattern however finds the particular object according to the command and invokes only that one.
It’s like there is a server having a lot of services to be given, and on Demand (or on command), it caters to that service for that particular client.
A classic example of this is a restaurant. A customer goes to restaurant and orders the food according to his/her choice. The waiter/ waitress takes the order (command, in this case) and hands it to the cook in the kitchen. The cook can make several types of food and so, he/she prepares the ordered item and hands it over to the waiter/waitress who in turn serves to the customer.
Let’s have a look at this example with Java code.
First thing is the Order. The order is made of command which the customer gives the waiter.
Order.java
package bahavioral.command; /** * Order.java * This is the command. The customer orders and * hands it to the waiter. */ public class Order { | ||
private String command; public Order(String command) { this.command = command; } | ||
}// End of class |
Waiter.java
package bahavioral.command; /** * A waiter is associated with multiple customers and multiple orders */ public class Waiter { | ||
public Food takeOrder(Customer cust, Order order) { Cook cook = new Cook(); Food food = cook.prepareOrder(order, this); return food; } | ||
}// End of class |
The waiter calls the prepareFood method of the cook who in turn cooks.
Cook.java
package bahavioral.command; public class Cook { | ||
public Food prepareOrder(Order order, Waiter waiter) { Food food = getCookedFood(order); return food; } public Food getCookedFood(Order order) { Food food = new Food(order); return food; } | ||
}// End of class |
The order is an object which depends on the command. The food item will change as soon as the command changes. This is loose-coupling between the client and the implementation.
Example : 2
Java reflection and the Command design pattern
01 Motivation. “Sometimes it is necessary to issue requests to objects without knowing anything about the operation being requested or the receiver of the request.” The Command design pattern suggests encapsulating (“wrapping”) in an object all (or some) of the following: an object, a method name, and some arguments. Java does not support “pointers to methods”, but its reflection capability will do nicely. The “command” is a black box to the “client”. All the client does is call “
02execute()
” on the opaque object. import java.lang.reflect.*;
public class CommandReflect {
private int state;
public CommandReflect( int in ) {
state = in;
}
public int addOne( Integer one ) {
return state + one.intValue();
}
public int addTwo( Integer one, Integer two ) {
return state + one.intValue() + two.intValue();
}
static public class Command {
private Object receiver; // the "encapsulated" object
private Method action; // the "pre-registered" request
private Object[] args; // the "pre-registered" arg list
public Command( Object obj, String methodName, Object[] arguments ) {
receiver = obj;
args = arguments;
Class cls = obj.getClass(); // get the object's "Class"
Class[] argTypes = new Class[args.length];
for (int i=0; i < args.length; i++) // get the "Class" for each
argTypes[i] = args[i].getClass(); // supplied argument
// get the "Method" data structure with the correct name and signature
try { action = cls.getMethod( methodName, argTypes ); }
catch( NoSuchMethodException e ) { System.out.println( e ); }
}
public Object execute() {
// in C++, you do something like --- return receiver->action( args );
try { return action.invoke( receiver, args ); }
catch( IllegalAccessException e ) { System.out.println( e ); }
catch( InvocationTargetException e ) { System.out.println( e ); }
return null;
} }
public static void main( String[] args ) {
CommandReflect[] objs = { new CommandReflect(1), new CommandReflect(2) };
System.out.print( "Normal call results: " );
System.out.print( objs[0].addOne( new Integer(3) ) + " " );
System.out.print( objs[1].addTwo( new Integer(4),
new Integer(5) ) + " " );
Command[] cmds = {
new Command( objs[0], "addOne", new Integer[] { new Integer(3) } ),
new Command( objs[1], "addTwo", new Integer[] { new Integer(4),
new Integer(5) } ) };
System.out.print( "\nReflection results: " );
for (int i=0; i < cmds.length; i++)
System.out.print( cmds[i].execute() + " " );
System.out.println();
} }
<br/>
Normal call results: 4 11 // 1 + 3 = 4 // 2 + 4 + 5 = 11 Reflection results: 4 11
No comments:
Post a Comment